allure-pytest and allure-pytest-bdd
Allure is a great tool for visualising your test cases in a neat interactive website.
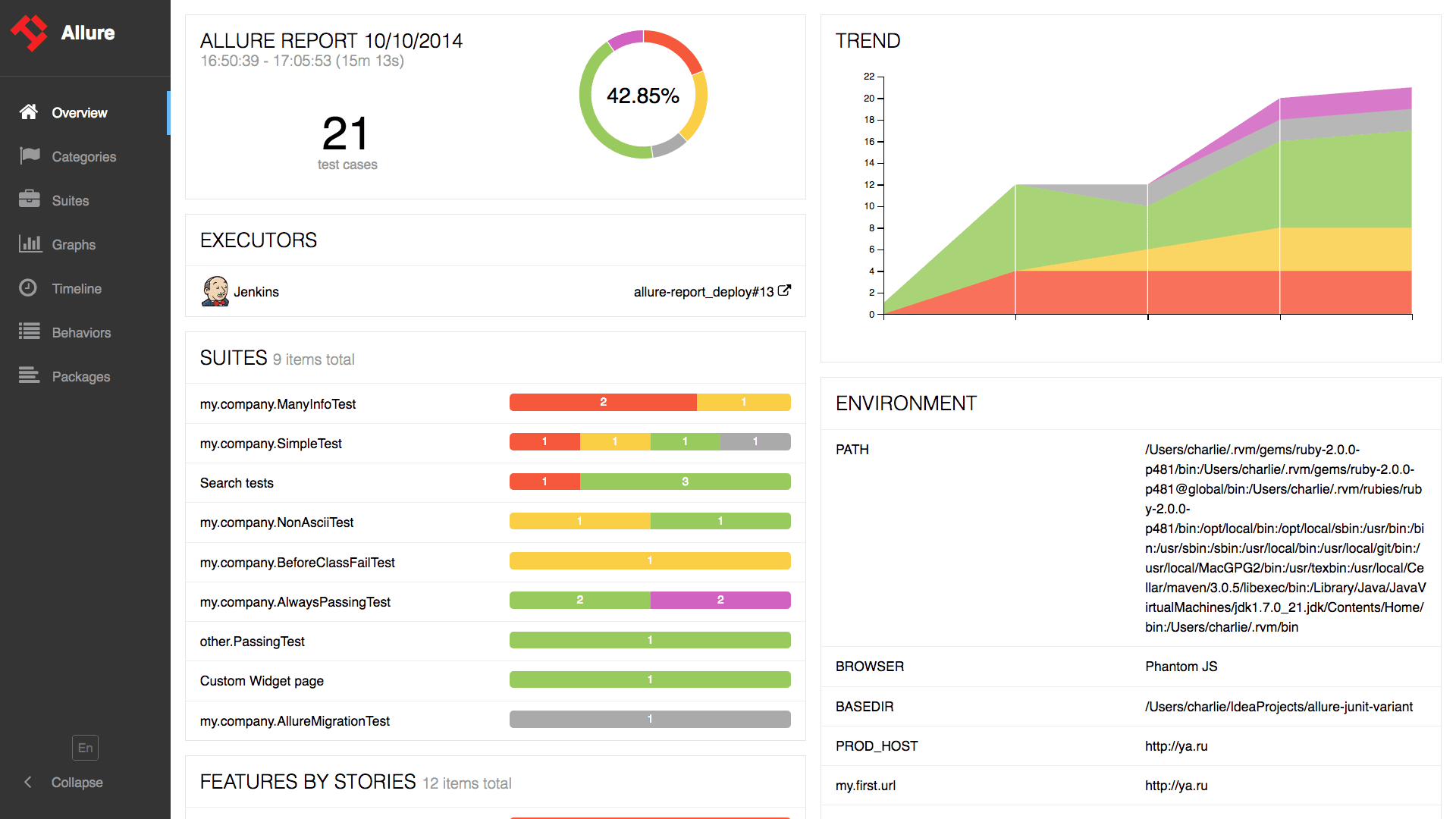
If you are using unit-tests as well as BDD style tests in your project, you will need two separate plugins for creating allure reports:
- allure-pytest: plugin for normal pytest unit-tests
- allure-pytest-bdd: a specific plugin for creating allure reports from BDD style tests
A different plugin is required for unit-tests and BDD tests because a slightly different allure report is created depending on the type of test.
You can install these two libraries using pip:
# install the allure libraries
pip install allure-pytest allure-pytest-bdd
However, these two libraries are incompatible with each other.
After installing both of these libraries, when you try and run your test suite you will be greeted with the following error – preventing you from running pytest:
ValueError: option names {'--alluredir'} already added
Fixing: ValueError: option names {'–alluredir'} already added
The error message is caused because both the allure-pytest
and allure-pytest-bdd
plugins are attempting to register the --alluredir
command-line option, resulting in a conflict.
To solve this issue and get both plugins working together seamlessly, we can utilise the -p
flag to disable or enable certain pytest plugins
at runtime.
For example, we can disable the conflicting plugin using -p
and no:
:
# run your unit-test report without allure-pytest-bdd plugin enabled
pytest -p no:allure_pytest_bdd --alluredir=allure-results
# run you bdd test report without allure-pytest plugin enabled
pytest -p no:allure_pytest --alluredir=allure-bdd-results
Note: Use underscores (
allure_pytest_bdd
) instead of hyphens (allure-pytest-bdd
) in the plugin name
This will solve the initial error. But I find it a little inconvenient.
You will have to disable at least one of the allure plugins each time you run your tests, regardless of whether you want to create a new allure report.
Pytest configuration
We can utilise the pytest configuration
to automatically add the -p
flag to disable plugins when we envoke pytest. This is achieved via the addopts
config parameter.
Pytest supports multiple different config files: pytest.ini
, pyproject.toml
, tox.ini
or setup.cfg
.
Using pytest.ini
as an example, we just add the following config
# pytest.ini
[pytest]
addopts =
-p no:allure_pytest
-p no:allure_pytest_bdd
This configuration will disable both allure plugins by default.
When we do want to create an allure report for our unit-tests or bdd tests we simply re-enable the plugin as required.
# create allure report for unit-tests (enable allure-pytest plugin)
pytest -p allure_pytest --alluredir=allure-results
# create allure report for bdd tests (enable allure-pytest-bdd plugin)
pytest -p allure_pytest_bdd --alluredir=allure-bdd-results
I find this much more convenient. In most cases when running my tests (e.g. debugging) I don’t need to create an allure-report and don’t need the plugin so automatically disabling the plugins via the config makes sense.
Further automation: Makefile
If you are familiar with using a Makefile
you can also create a specific command for running the test suite producing an allure report.
For example:
# Makefile
create-allure-bdd-report:
pytest -p allure_pytest_bdd --alluredir=allure-bdd-results
allure generate --clean --output allure-bdd-report allure-bdd-results
# run the test suite and create allure report for the bdd tests
make create-allure-bdd-report
đź’» Code examples can be found in the e4ds-snippets GitHub repository
Happy coding!
Further Reading
- Top tips for using PyTest
- How to mock sending SMTP emails using PyTest
- Google Search Console API with Python
- Deploying Dremio on Google Cloud
- Gitmoji: Add Emojis to Your Git Commit Messages!
- Five Tips to Elevate the Readability of your Python Code
- Do Programmers Need to be able to Type Fast?
- How to Manage Multiple Git Accounts on the Same Machine